It’s useful to be able to read and plot serial data in real time (for example, you might want to monitor the output of a laser scanner or IMU). While this is a trivial task in MATLAB or LabVIEW, I wondered if there was a low effort way to do it for free.
Python Serial.readuntil - 4 examples found. These are the top rated real world Python examples of serial.Serial.readuntil extracted from open source projects. You can rate examples to help us improve the quality of examples. In this section, we will focus on sending data from the Arduino to the computer over a serial connection, and then plotting it with Python.We will use the data from a potentiometer as an example for the code below since it involves only a simple analogRead. In addition, I have limited the scope of this post to just sending float and int data types since these 2 data types will be sufficient. Python Serial.write - 30 examples found. These are the top rated real world Python examples of serial.Serial.write extracted from open source projects. You can rate examples to help us improve the quality of examples. ReadString will read characters from the serial (or other Stream) device until a timeout occurs. That timeout is, by default, 1 second. That timeout is, by default, 1 second. It is only appropriate to use readString if your data is arriving in chunks with a minimum time between each chunk. API¶ class periphery.Serial (devpath, baudrate, databits=8, parity='none', stopbits=1, xonxoff=False, rtscts=False) source ¶. Bases: object Instantiate a Serial object and open the tty device at the specified path with the specified baudrate, and the defaults of 8 data bits, no parity, 1 stop bit, no software flow control (xonxoff), and no hardware flow control (rtscts).
Python Serial - 30 examples found. These are the top rated real world Python examples of serial.Serial extracted from open source projects. You can rate examples to help us improve the quality of examples. If it ain't broke, I just haven't gotten to it yet. OS: Windows 10, openSuse 42.3, freeBSD 11, Raspian 'Stretch' Python 3.6.5, IDE: PyCharm 2018 Community Edition.
I’ve known for a while that Python has an easy-to-use serial library, but I wasn’t sure what kinds of plotting/graphing options might exist for Python. A quick search turned up Matplotlib – a MATLAB-like plotting API for Python. As it turns out, Matplotlib includes an animation API and a function called FuncAnimation, which can be used to animate data over time (or update a graph with some sensor data over time).
I’m using this page to document my attempt(s) to use Matplotlib to create a real time graph of data read from a serial port. For a proper introduction to Matplotlib, I’d recommend sentdex’s Matplotlib video series.

Items used
- Laptop or PC running Ubuntu 18
- Python 3.x
- Matplotlib
- pyserial
- Arduino (or any programmable device with a serial port)
Installing matplotlib and pyserial on Ubuntu 18
Python Serial Read Timeout Example
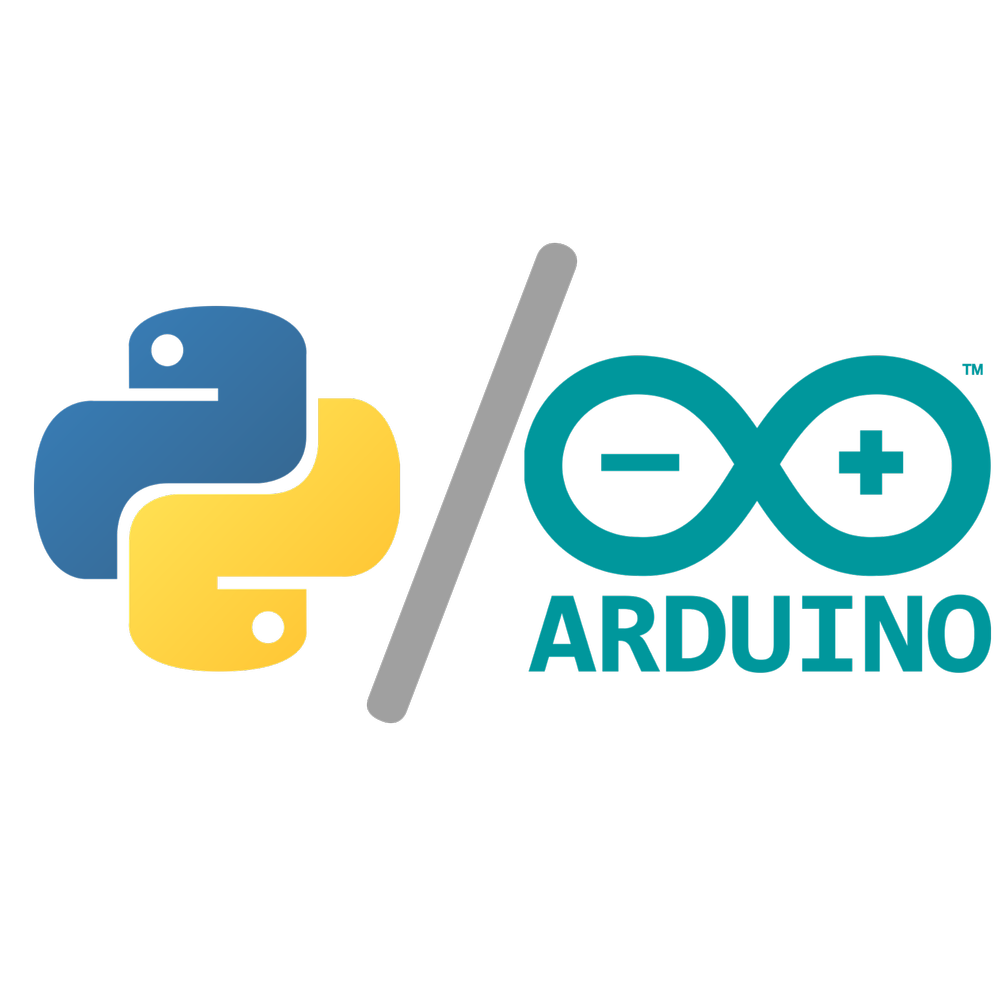
Generating some fake serial data with an Arduino

To test my code, I used an Arduino to put some data on the serial port. In the example code below, the arduino simulates a coin toss using the function random.
Python Serial Read Example
On each iteration of the main loop, a random integer between 0 and 1 is generated, and the relative frequency of getting one side of the coin or the other is updated. This data is then sent to the serial port as comma delimitted line, where the termination character is ‘/n’. That is, the serial data looks like this:
The idea is that I’m putting some data on a serial port over time, and now I can write a python script to read and plot it.
Python Serial Port Read Example
Creating the real time plot
References
- https://pythonhosted.org/pyserial/shortintro.html
- https://www.youtube.com/watch?v=ZmYPzESC5YY
- https://matplotlib.org/
- https://matplotlib.org/api/_as_gen/matplotlib.animation.FuncAnimation.html#matplotlib.animation.FuncAnimation